What is IntentKit?
IntentKit is an open-source iOS library that makes it easier to link to other apps. It's sort of like Android's Intents or Windows Phone's Contracts.
Why should I use it?

People like choices.
IntentKit makes it easy for users to use their favorite third-party apps instead of Apple's defaults for tasks like browsing the web, mapping, and sending mail.

Readable code matters.
Constructing URLs by hand is ugly. IntentKit replaces string manipulation with a clean, semantic API, making your code easier to work with.

Your time is valuable.
IntentKit is maintained by the community, so you don't have to worry about manually fixing your app when a third-party app changes their URL scheme.
What does it look like?
IntentKit has an example project you can use to see it in action. Assuming you have Cocoapods installed, you can take it for a spin by typing the following command at a terminal prompt:
$ pod try IntentKit
Otherwise, follow the instructions on GitHub to get the example project up and running.
How can I get it?
IntenKit is easiest to install using CocoaPods. If your project already uses CocoaPods, it's as easy as adding it to your Podfile.
pod "IntentKit"
It's also possible to use IntentKit without CocoaPods.
How do I use it?
To use IntentKit, you just need to instantiate a handler object, give it an action to perform, and tell the resulting presenter object to present itself. Handlers exist for common tasks such as opening web sites, displaying maps, and interacting with Twitter.
Here's how you'd compose an email:
INKMailHandler *mailHandler = [[INKMailHandler alloc] init]; [[mailHandler sendMailTo:@"steve@apple.com"] presentModally];
If the user doesn't have any third-party email clients installed, this will open up an inline MFMailComposeController
. If the user has other mail clients, this will display a modal sheet giving them a choice of all currently-installed apps. All that from two lines of code!
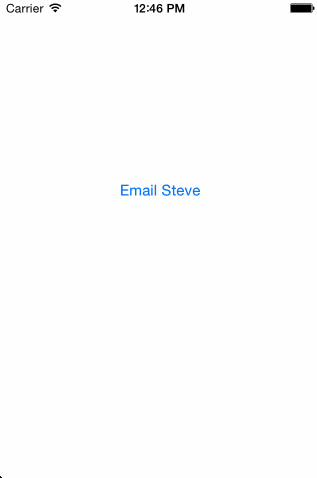
The real power of IntentKit shines through when you start dealing with more complex actions, like opening up turn-by-turn directions in a mapping application.
INKMapsHandler *mapsHandler = [[INKMapsHandler alloc] init]; mapsHandler.center = CLLocationCoordinate2DMake(42.523, -73.544); mapsHandler.zoom = 14; [mapsHandler directionsFrom:@"Washington Square Park" to:@"Lincoln Center"];
If you don't want to bug the user by asking them which app to use, IntentKit can be more hands-off. It can be configured to use system defaults and instead provide a configuration screen within your app's settings page to let power users change their defaults at their own leisure.
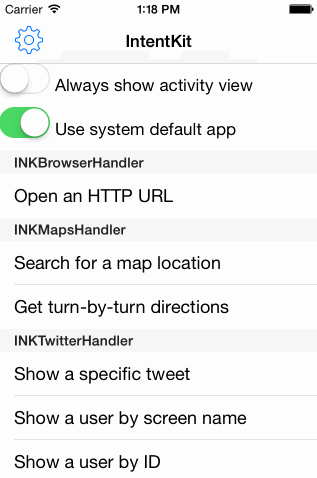
IntentKit has lots of other features as well, such as a built-in in-app browser to display web sites. More complete usage instructions are available on GitHub. An up-to-date list of available handlers can be found on CocoaDocs.
How Do I Learn About Changes to IntentKit?
IntentKit is under active development, so new improvements happen rather frequently. Follow @IntentKit on Twitter to keep up-to-date on the latest news and releases.
Add Your Own URL Scheme
IntentKit might not yet support some of your favorite applications. Fortunately, adding support for a new URL scheme is easy. In most cases, it doesn't require writing any code!
Just fork the IntentKit GitHub repo, create a property list detailing a URL scheme, drop in some icons, and send a pull request. For detailed instructions, check out GitHub.